Introduction
The Beacon web apps allow you to add some of your customizations. For example, your plugin code can listen to user events and process custom code based on your requirements.
The user events are triggered by user interactions with the Beacon app each time a user sign in, register, or sign out.
Use cases
The following are appropriate use cases for the user events.
- With the help of the user events, you can retrieve claims of the account tokens.
- You can also use the user events to save login or registration data so your user can log into other applications such as PigeonHole.
Events
The following events can be used in your plugin code to control the user events.
Event | Details |
---|---|
userDidSignIn |
Called when the sign in process is complete with success. |
userDidRegister |
Called when the register process is complete with success. |
userDidSignOut |
Called before the logout request is done to Beacon APIs. |
Event data
When listening to user events, you can display the event data which is available for use in your custom code. Here is the example of this data:
Data element | Type |
---|---|
account_token |
String |
user_name |
String |
user_email |
String |
claims |
Object |
Using the user events
There are three types of user events in your Beacon application that you can use. User events are implemented to retrieve users' data when signing in, registering, or signing out.
User did sign in
The following image shows an example of the workflow for the
userDidSignIn
event:
The following image shows an example of the data retrieved with the
userDidSignIn
event:
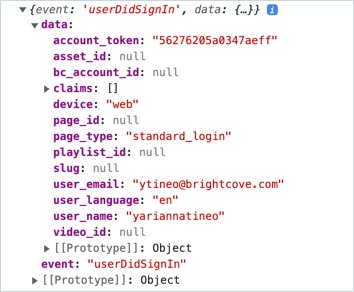
User did register
The following image shows an example of the workflow for the
userDidRegister
event:
The following image shows an example of the data retrieved with the
userDidRegister
event:
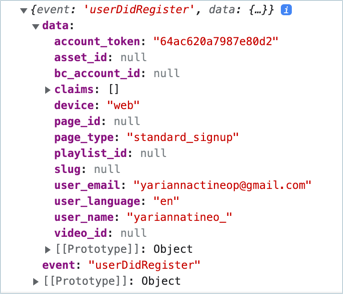
User did sign out
The following image shows an example of the workflow for the
userDidSignOut
event:
The following image shows an example of the data retrieved with the
userDidSignOut
event:
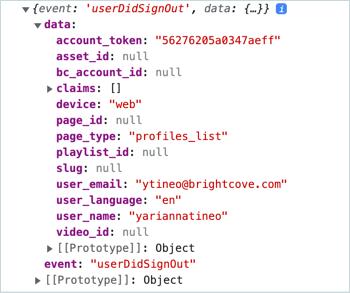
Module implementation
The following shows how the user events are implemented using JavaScript modules. Of course, you may implement your JavaScript in the manner of your choice. Further details on this module implementation can be found in the Implementing OTT Plugin Code Using Modules document.
index.js
Below is the JavaScript code used for the user events example:
window.addEventListener("message", (event) => {
switch (event.data.event) {
case 'userDidSignIn':
console.log(event.data)
break;
case 'userDidRegister':
console.log(event.data)
break;
case 'userDidSignOut':
console.log(event.data)
break;
}
});